https://github.com/SeeedDocument/Seeed-WiKi/blob/master/docs/Grove-Gas_Sensor-O2.md
The Seeed Studio O2 sensor is a nice Oxygen sensor. It works up to 25% Oxygen concentration and is easy to use in an Arduino project.
http://wiki.seeedstudio.com/Grove-Gas_Sensor-O2/
Wiring Up the O2 Sensor to Arduino
If you look on the back of the sensor there are only 3 wires used. VCC, GND, and a SIG. The NC pin is “Not Connected”
Wire the
GND -> Arduino ground
VCC -> Arduino 3.3V (Looks like it may also accept 5V)
SIG -> A5 (Analog 5)
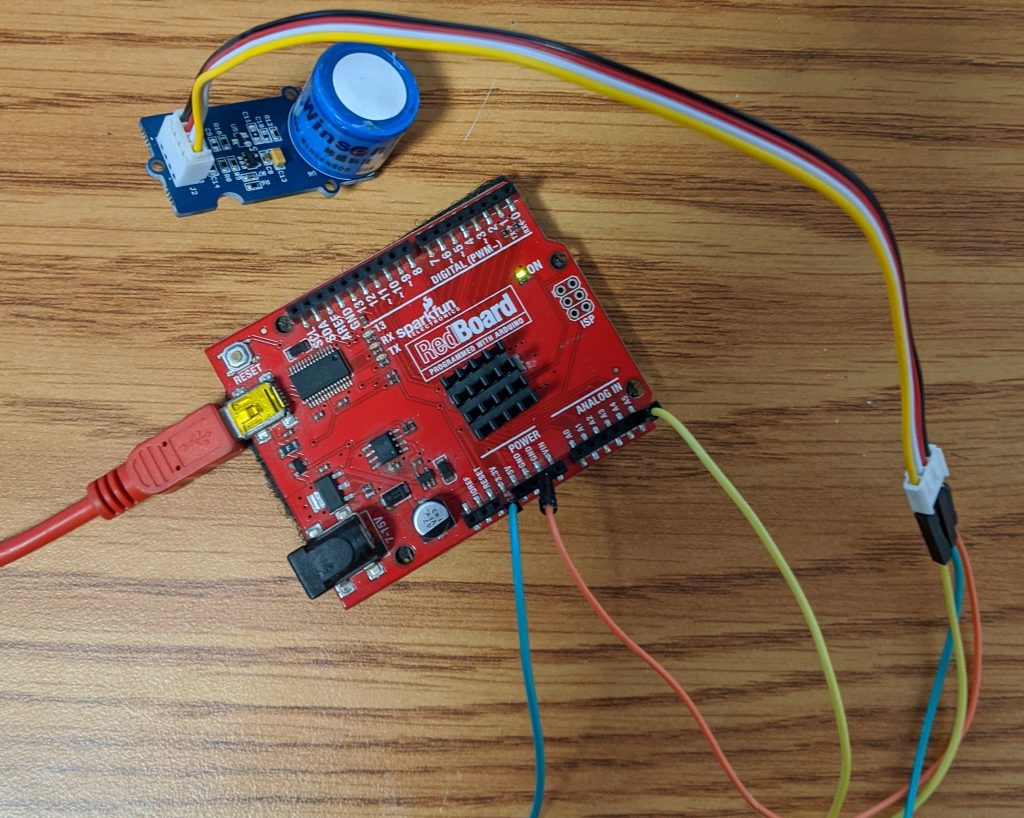
Code to read O2 Sensor
Copy and paste the following code into the Arduino IDE
// Grove - Gas Sensor(O2) test code // Note: // 1. It need about about 5-10 minutes to preheat the sensor // 2. modify VRefer if needed const float VRefer = 3.3; // voltage of adc reference const int pinAdc = A5; void setup() { // put your setup code here, to run once: Serial.begin(9600); Serial.println("Grove - Gas Sensor Test Code..."); } void loop() { // put your main code here, to run repeatedly: float Vout =0; Serial.print("Vout ="); Vout = readO2Vout(); Serial.print(Vout); Serial.print(" V, Concentration of O2 is "); Serial.println(readConcentration()); delay(500); } float readO2Vout() { long sum = 0; for(int i=0; i<32; i++) { sum += analogRead(pinAdc); } sum >>= 5; float MeasuredVout = sum * (VRefer / 1023.0); return MeasuredVout; } float readConcentration() { // Vout samples are with reference to 3.3V float MeasuredVout = readO2Vout(); //float Concentration = FmultiMap(MeasuredVout, VoutArray,O2ConArray, 6); //when its output voltage is 2.0V, float Concentration = MeasuredVout * 0.21 / 2.0; float Concentration_Percentage=Concentration*100; return Concentration_Percentage; }
Upload and Launch the Serial Monitor
Tools -> Serial Monitor
or
Ctrl + Shift + M
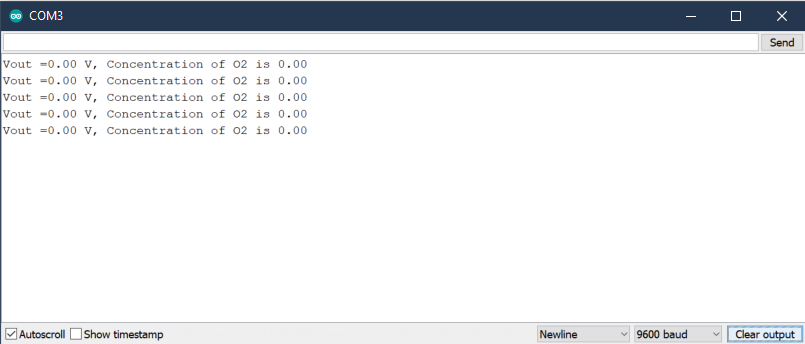