Below is a code example for creating a basic object and using a function to calculate the fuel economy.
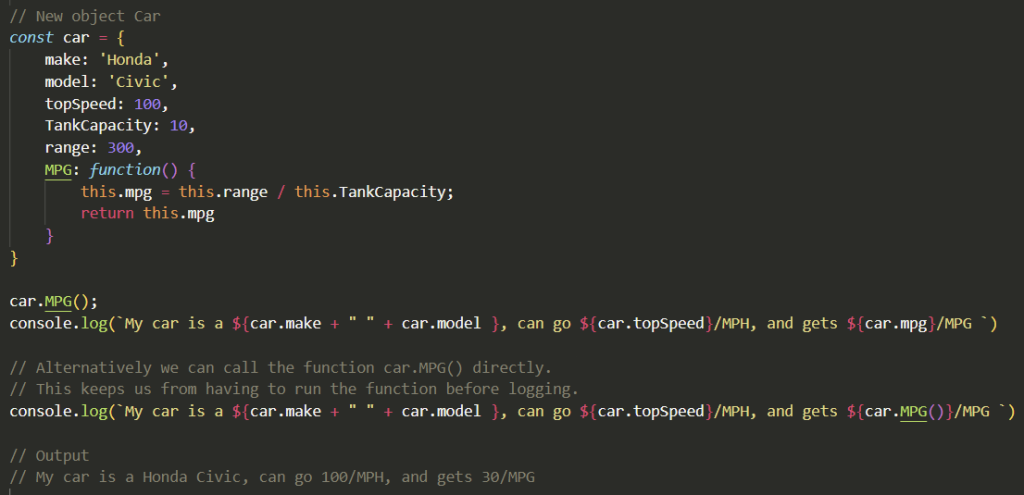
// New object Car
const car = {
make: 'Honda',
model: 'Civic',
topSpeed: 100,
tankCapacity: 10,
range: 300,
MPG: function() {
this.mpg = this.range / this.tankCapacity;
return this.mpg
}
}
car.MPG(); // We need to call this to calculate the MPG, otherwise we get undefined
console.log(`My car is a ${car.make + " " + car.model }, can go ${car.topSpeed}/MPH, and gets ${car.mpg}/MPG `)
// Alternatively we can call the function car.MPG() directly.
// This keeps us from having to run the function before logging.
console.log(`My car is a ${car.make + " " + car.model }, can go ${car.topSpeed}/MPH, and gets ${car.MPG()}/MPG `)